Cesium中的实体Entity
包括Boxes、
1 | //这两行函数针对每一个代码块的代码都需要加上才能运行成功;分别放置在首尾位置 |
Boxes
蓝色的立方体
1 | //添加一个蓝色的立方体 |
具有黑色边框的立方体
1 | //具有黑色边界的红色立方体 |
黄色立体边框
1 |
|
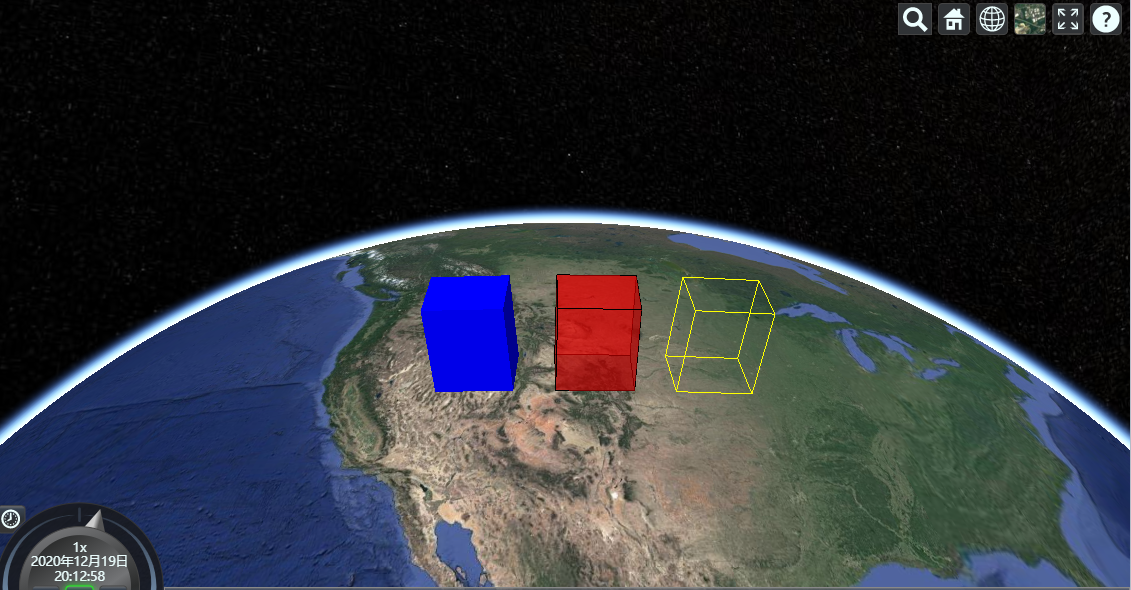
Circles and Ellipses
带有边界的绿色圆
1 | //Green circle at height with outline |
位于地球表面的红色椭圆
1 | //Red ellipse on surface |
蓝色柱体,旋转45°
1 | var blueEllipse = viewer.entities.add({ |
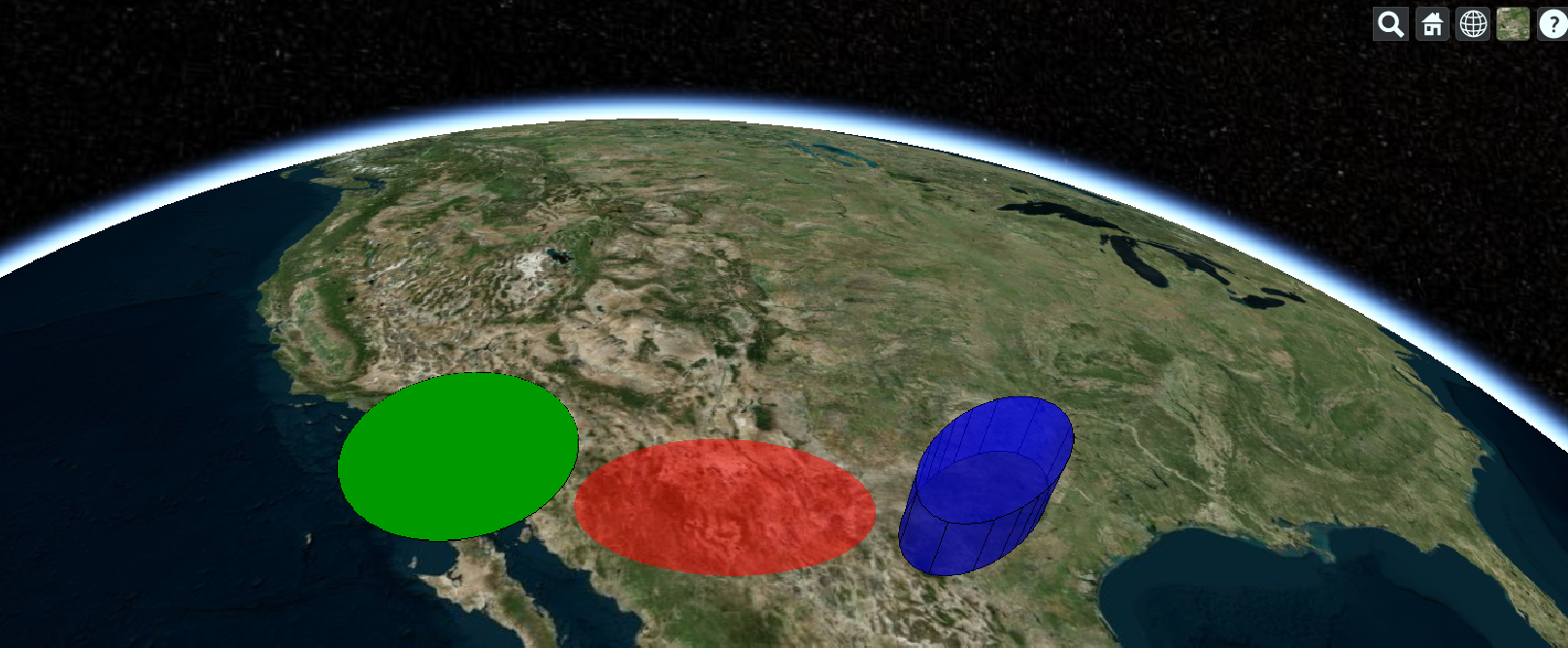
corridor
1 | var redCorridor = viewer.entities.add({ |
1 | var greenCorridor = viewer.entities.add({ |
1 | var blueCorridor = viewer.entities.add({ |
Cylinder and Cones
1 | var greenCylinder = viewer.entities.add({ |
1 | var redCone = viewer.entities.add({ |
Polygon
红色多边形
1 | var redPolygon = viewer.entities.add({ |
绿色延伸多边形
1 | var greenPolygon = viewer.entities.add({ |
带有每个位置高度和轮廓的橙色多边形
每个点的高度不一样
1 | var orangePolygon = viewer.entities.add({ |
中空的蓝色多边形
1 | var bluePolygon = viewer.entities.add({ |
带有每个位置高度和轮廓的青色垂直多边形
每个点的参数有三个(分别是经度、纬度、高度)
1 | var cyanPolygon = viewer.entities.add({ |
有轮廓的菱形线的紫色多边形
outline边框线的类型是虚线,而非实线
1 | var purplePolygonUsingRhumbLines = viewer.entities.add({ |
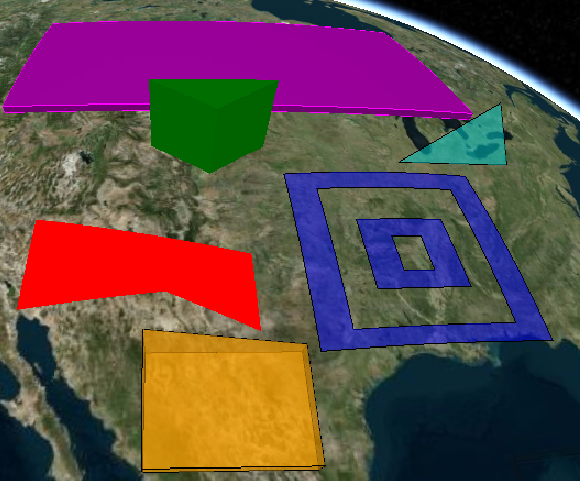
PolyLines
arcType:获取或设置ArcType属性,该属性指定线段应该是大圆弧、直角线还是线性连接的,ArcType定义连接顶点应采用的路径。
- 有三个路径NONE、GEODESIC、RHUMB。
- RHUMB:恒向线是始终与经线保持相同的角度;
clampToGround:获取或设置布尔属性,该布尔属性指定是否应将折线固定在地面上。默认为false。
position:位置
width:宽度
material:这个参数可以通过调用函数来丰富内容,函数的种类包括多种,可以是虚线、发光的线等等
- 函数名的例子:PolylineGlowMaterialProperty,Glow替换掉其他的即可;
在地形上的红色线
1 | var redLine = viewer.entities.add({ |
绿色恒向线
1 | var greenRhumbLine = viewer.entities.add({ |
在表面发光的蓝线
- PolylineGlowMaterialProperty该函数有三个参数,分别是
- color:颜色
- glowPower:用于指定发光强度
- taperPower:指定渐缩效果的强度,以占总线长的百分比表示。如果为1.0或更高,则不使用锥度效果。
1 | var glowingLine = viewer.entities.add({ |
带有黑色轮廓的橙色线,具有一定的高度并且沿着表面
- PolylineOutlineMaterialProperty函数有三个参数:color颜色、outlineColor轮廓颜色、outlineWidth轮廓宽度
1 | var orangeOutlined = viewer.entities.add({ |
具有一定高度的紫色的直箭头
- PolylineArrowMaterialProperty只有一个color函数
1 | var purpleArrow = viewer.entities.add({ |
蓝色虚线
1 | var dashedLine = viewer.entities.add({ |
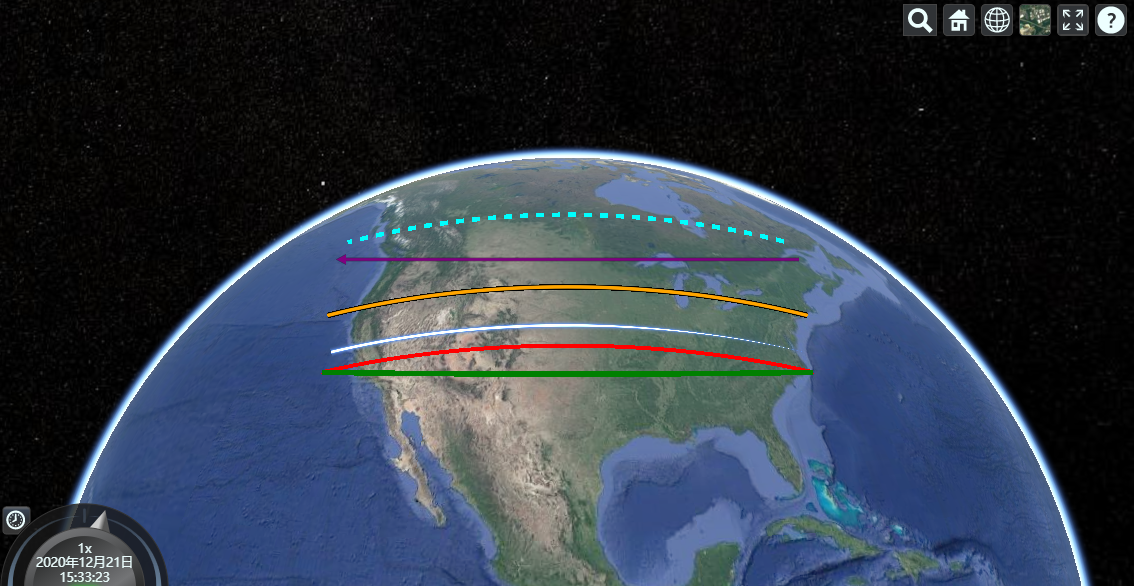
Polyline Volumes
待定
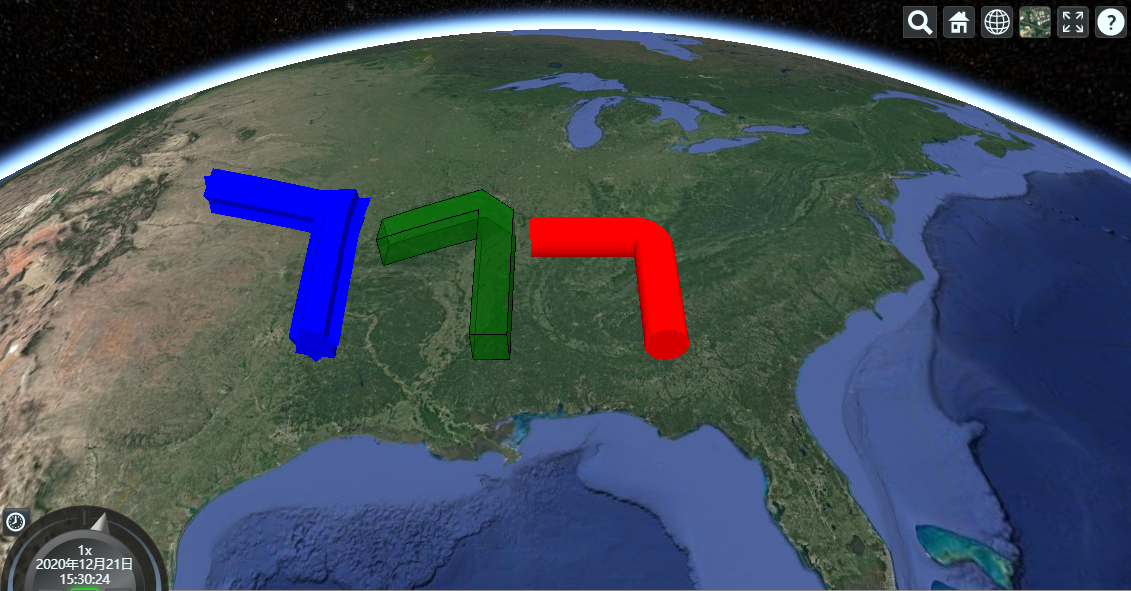
Rectangle
红色矩形
1 | var redRectangle = viewer.entities.add({ |
绿色半透明,旋转,和挤压矩形具有高度和轮廓
1 | var greenRectangle = viewer.entities.add({ |
1 | var rotation = Cesium.Math.toRadians(30); |
旋转矩形
1 | viewer.entities.add({ |
Spheres and Ellipsoids
- 确定一个位置,然后增加一个半径
1 | var blueEllipsoid = viewer.entities.add({ |
1 | var redSphere = viewer.entities.add({ |
1 | var outlineOnly = viewer.entities.add({ |
Walls
1 | var redWall = viewer.entities.add({ |
1 | var greenWall = viewer.entities.add({ |
1 | var blueWall = viewer.entities.add({ |